Mobile game get player inputs by the buttons, each with a specific function, or by its attribute like tap or long tap. The former is simple. It just calls each public method. However, the latter case need manage flags as many as the number of commands, then it`s likely to complicate. The corutine make this easier.
Environment: Unity 2018.4.6f
Get a single command
When we get a input in Unity, we can use * EventTrigger and Input API *. These have several methods and we select one depending on the situation.
In this case, we only need to write a process in the method. Even if we use two or more button, we just prepare the processing for each one.S
Use EventTrigger
When player tap on the screen, EventTrigger invoke a public method. All we need to prepare is the process calling at tap.
EventTrigger has the two way to use. One is adding its component to a object in Unity Editor. It gets a input via the object, so it also need Raycast Target. Another one is calling Event Trigger directly. In this case, the EventTrigger must be inherited.
using UnityEngine;
public class SingleTap : MonoBehaviour {
public void PressButton(){
Debug.Log("Punch");
}
public void ReleaseButton(){
Debug.Log("Kick");
}
}
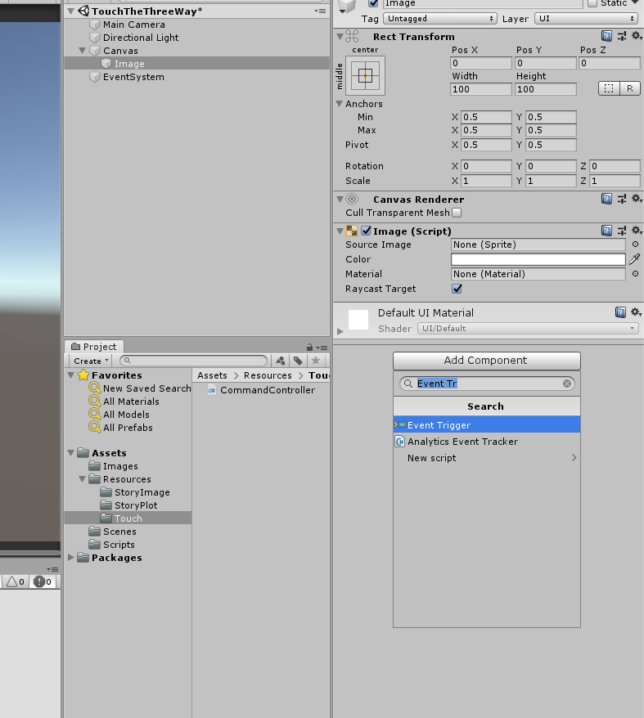
It can call public method like Button. Check the document about when is each trigger.
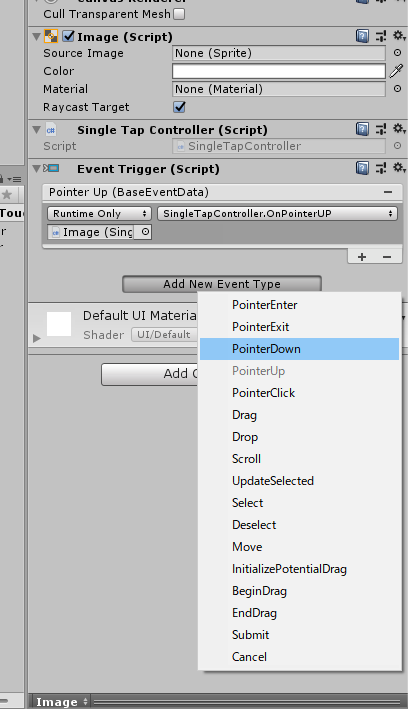
See the document about how to call an Event directly.
Use Input method
We can also use Input.GetMouseButton to get touch. Usually, we use it with if statement in Update. It return true when player taps somewhere on the screen. When more than one is enumerated, the process may be called consecutively.
If a project needs to recognize more than one finger at a time, it need to use Input.GetTouch instead of Input.GetMouseButton.
using UnityEngine;
public class SingleClick : MonoBehaviour{
void Update(){
if (Input.GetMouseButton(0)){
Debug.Log("Punch");
}
}
}
Get three type inputs by a button
To identify tap, double tap, and long tap, a flag is switched after the first tap. When this is implemented in Update, the nest tends to become deeper.
Corutine can make it readability and scalability. That just start it after the first input.Note the first one is a long tap.
using System.Collections;
using UnityEngine;
public class ThreeWayTap : MonoBehaviour {
public int touchType { get; private set; }
//Can change the interval with this.
float inputLimit = 0.3f;
//Set to PointerDown Event / or ues EventTrigger method.
public void PressButton() {
if (touchType <= 0) {
StartCoroutine(CountWaitTime());
touchType = 1;
} else {
touchType = 2;
}
}
//Set to PointerUp Event.
public void ReleaseButton() {
if (touchType == 1) {
touchType = 3;
}
}
IEnumerator CountWaitTime() {
yield return new WaitForSeconds(inputLimit);
switch (touchType){
case 1:
Debug.Log("LongTap");
break;
case 2:
//Tap, Lift, Tap again
Debug.Log("DoubleTap");
break;
case 3:
//Tap, Lift
Debug.Log("SingleTap");
break;
}
//Initialize
touchType = 0;
}
}
Recognize multiple tap
As for multiple tap like two or more one, we can implement it by the same way. This example extends the time to accept inputs on new tap.
using System.Collections;
using UnityEngine;
public class MultipleTap : MonoBehaviour {
public int touchCount { get; private set; }
float inputLimit = 0.3f;
float additionalTime;
public void PressButton() {
if (touchCount <= 0) {
StartCoroutine(CountWaitTime());
touchCount = 1;
}else{
//Can change the additional limit here
//variable one's ex. 0.2f - (0.1f * touchcount)
additionalTime += 0.1f;
touchCount ++;
}
}
IEnumerator CountWaitTime() {
yield return new WaitForSeconds(inputLimit);
while(additionalTime < 0f){
float preLimit = additionalTime;
yield return new WaitForSeconds(additionalTime);
//For getting out of the loop.
//If player press a button by the limit, this loop again.
additionalTime -= preLimit;
}
switch (touchCount){
case 1:
Debug.Log("SingleTap");
break;
default:
Debug.Log(touchCount + "Tap");
break;
}
touchCount = 0;
//This may be subtracted enough, but for the standardization.
additionalTime = 0.0f;
}
}
References
Unity – Scripting API: EventTrigger
Unity – Scripting API: Input